C Program To Implement Dictionary Using Hashing Out
Prerequisite –Hashing Introduction, Implementing our Own Hash Table with Separate Chaining in Java Miss pooja songs download 2014.
C Program To Implement Dictionary Using Hashing It Out Medium password security using SHA algorithms. The SHA (Secure Hash Algorithm) is a family of cryptographic hash functions.
In Open Addressing, all elements are stored in the hash table itself. So at any point, size of table must be greater than or equal to total number of keys (Note that we can increase table size by copying old data if needed).
- Insert(k) – Keep probing until an empty slot is found. Once an empty slot is found, insert k.
- Search(k) – Keep probing until slot’s key doesn’t become equal to k or an empty slot is reached.
- Delete(k) – Delete operation is interesting. If we simply delete a key, then search may fail. So slots of deleted keys are marked specially as “deleted”.
Here, to mark a node deleted we have used dummy node with key and value -1.
Insert can insert an item in a deleted slot, but search doesn’t stop at a deleted slot.
Dictionary
The entire process ensures that for any key, we get an integer position within the size of the Hash Table to insert the corresponding value.
So the process is simple, user gives a (key, value) pair set as input and based on the value generated by hash function an index is generated to where the value corresponding to the particular key is stored. So whenever we need to fetch a value corresponding to a key that is just O(1).
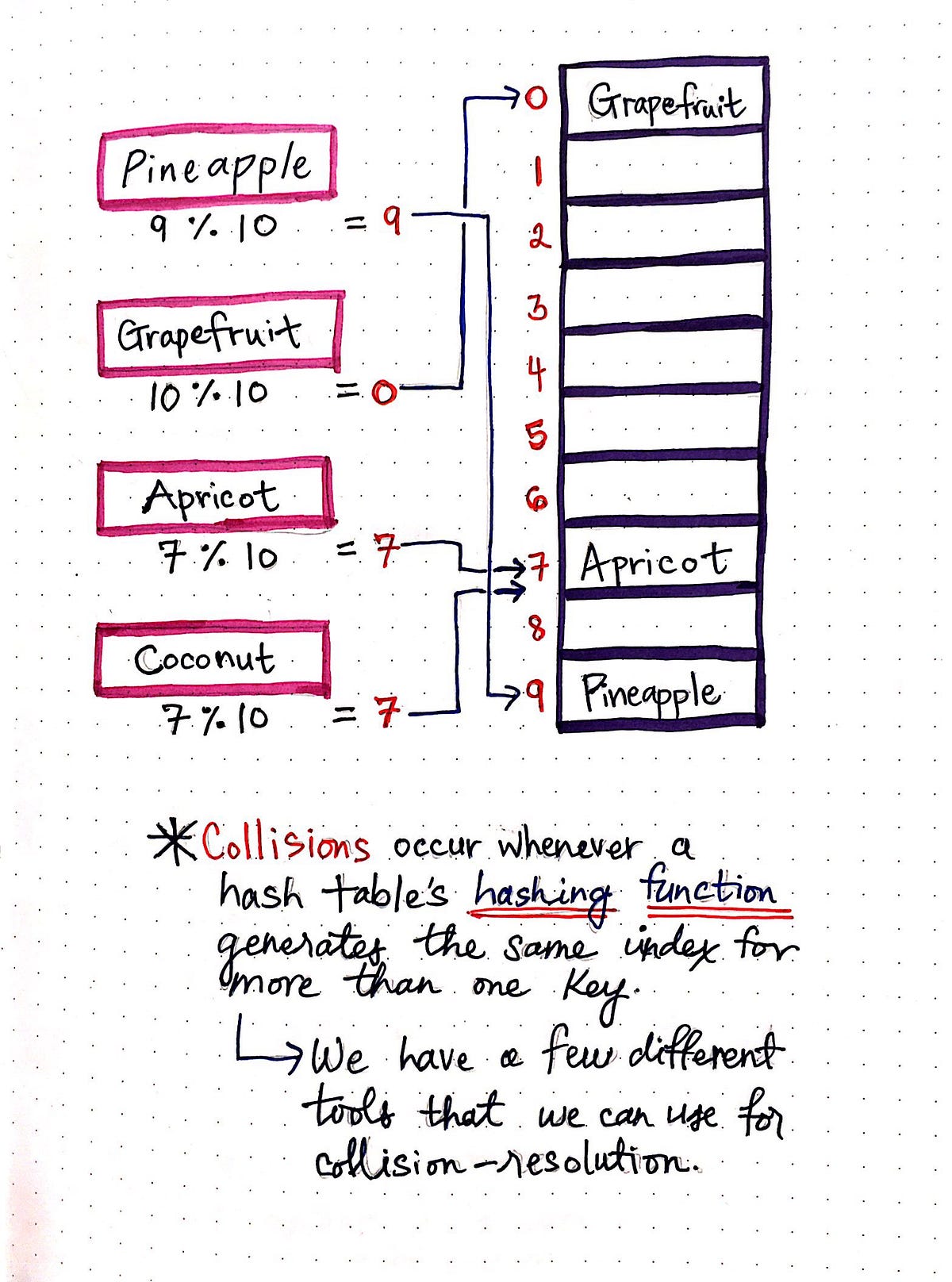
Code –
using namespace std; //template for generic type class HashNode public : K key; //Constructor of hashnode { this ->key = key; }; //template for generic type class HashMap //hash element array int capacity; int size; HashNode<K,V> *dummy; public : { capacity = 20; arr = new HashNode<K,V>*[capacity]; //Initialise all elements of array as NULL arr[i] = NULL; //dummy node with value and key -1 } // for a key { } //Function to add key value pair { HashNode<K,V> *temp = new HashNode<K,V>(key, value); // Apply hash function to find index for given key while (arr[hashIndex] != NULL && arr[hashIndex]->key != key { hashIndex %= capacity; //if new node to be inserted increase the current size if (arr[hashIndex] NULL arr[hashIndex]->key -1) arr[hashIndex] = temp; V deleteNode( int key) // Apply hash function to find index for given key while (arr[hashIndex] != NULL) //if node found { arr[hashIndex] = dummy; // Reduce size return temp->value; hashIndex++; return NULL; V get( int key) // Apply hash function to find index for given key int counter=0; while (arr[hashIndex] != NULL) if (counter++>capacity) //to avoid infinite loop //if node found return its value return arr[hashIndex]->value; hashIndex %= capacity; return NULL; int sizeofMap() return size; bool isEmpty() return size 0; void display() for ( int i=0 ; i<capacity ; i++) if (arr[i] != NULL && arr[i]->key != -1) << ' value = ' << arr[i]->value << endl; } int main() HashMap< int , int > *h = new HashMap< int , int >; h->insertNode(2,2); h->display(); cout << h->deleteNode(2) << endl; cout << h->isEmpty() << endl; } |
Output –
This article is contributed by Chhavi. If you like GeeksforGeeks and would like to contribute, you can also write an article using contribute.geeksforgeeks.org or mail your article to contribute@geeksforgeeks.org. See your article appearing on the GeeksforGeeks main page and help other Geeks.

Please write comments if you find anything incorrect, or you want to share more information about the topic discussed above.